in this article, we will talk about how to build SEO-friendly single-page apps (SPA) by adding dynamic META TAGS and dynamic page Title with Angular 11 with example.
Let’s now learn how to add dynamic meta tags and change page title to Angular 11 Application. We will use @angular/platform-browser to get or set the Title and Meta tags in our Angular application.
Table of Contents
How to add Angular Universal to existing project
Adding Angular Universal(SSR) in an existing application is as simple as these 2 steps:
- Add Angular Universal package ng add @nguniversal/express-engine
- Build application npm run build:ssr && npm run serve:ssr
How to add dynamic meta tags (SEO) in Angular Universal
Open the app.component.ts file and import “Title” and “Meta” services from “@angular/platform-browser” and inject both services in the component constructor as follows.
import { Component, OnInit } from '@angular/core';
import { Title, Meta } from '@angular/platform-browser';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements OnInit {
constructor(
private _http: HttpClient,
private _titleService: Title,
private _metaService: Meta) {
}
}
Next, we can mock any API JSON response data inside an assets folder. Create an empty JSON file called app-component.json with the following code.
[
{
"name": "keywords",
"content": "Angular 11, Universal, Dynamic Tags Example"
},
{
"name": "description",
"content": "Angular 11 Universal Example"
}
]
Next, get meta tags data from the server( in this demo we are fetching data from the assets folder)
ngOnInit() {
this.getMetaTagsData();
}
// get component meta data
getMetaTagsData() {
this._http.get<MetaDefinition[]>("/assets/metatags/app-component.json").subscribe(res => {
this.setMetaTags(res);
})
}
// set meta data
setMetaTags(tags: MetaDefinition[]) {
this._metaService.addTags(tags)
}
Next, build and serve the Angular application using the following commands.
npm run build:ssr
npm run serve:ssr
Next, run the server command, the following output will be displayed.
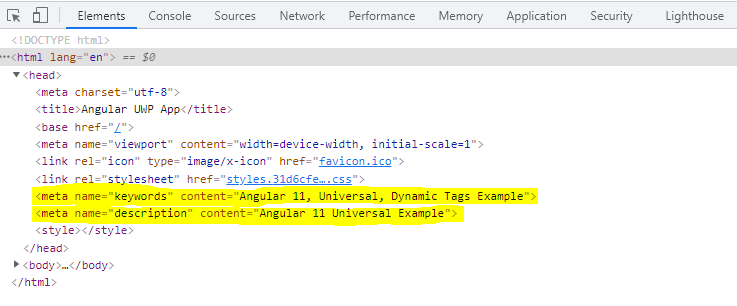
How to add dynamic page title in Angular Universal
To change the title dynamically, we will use the Title service.
Next, we can mock any API JSON response data inside an assets folder app-component-title-admin.json file with the following code.
{
"title": "Admin Dashboard"
}
Let’s see the simple example of the setTitle method of the Title service.
ngOnInit() {
this.getPageTitleData();
}
// get component title
getPageTitleData() {
this._http.get<ITitle>("http://localhost:4000/assets/titles/app-component-title-admin.json").subscribe(res => {
this.setPageTile(res.title);
})
}
// set page title
setPageTile(title: string) {
this._titleService.setTitle(title);
}
Next, run the server command, the following output will be displayed.

Further Reading
This post is a basic SEO-friendly single-page apps introduction to Angular Universal. For a more detailed guide, have a look at this page.
Source code available for download
The source code is available on GitHub for the Angular material 13 image upload.
URL:https://github.com/decodedscript/angular-material-13-image-upload