In Angular, the Date Pipe is great, but if you want to be able to support multiple language in your web app you might want to create your own custom pipe that support more the one date format (with some input parameter). in this article, I will show how to create custom localized Date Pipe, how to use TranslationService of @ngx-translate package and then we will add them in our application modules.
Table of Contents
Implementing Custom (localized) Date Pipe with TranslationService
Let’s start coding, I have created a date pipe “localizedDate” in my Angular project. this is an example of how the pipe can look is as follows.
import { DatePipe } from '@angular/common';
import { Pipe, PipeTransform } from '@angular/core';
import { TranslateService } from '@ngx-translate/core';
@Pipe({
name: 'localizedDate',
pure: false
})
export class LocalizedDatePipe implements PipeTransform {
constructor(
private translateService: TranslateService,
private datePipe: DatePipe
) {}
//pattern parameter default format is ‘mediumDate’.
transform(value: any, pattern: string = 'mediumDate'): any {
if (value)
return this.datePipe.transform(
value,
pattern,
undefined,
this.translateService.currentLang
);
}
}
Define the default language for the application
Once you’ve Created your custom Localized Date Pipe, you can import it in appModule.ts. and set the default language of your application using translate.setDefaultLang(‘en’).
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { CommonModule, DatePipe, registerLocaleData } from '@angular/common';
import { AppComponent } from './app.component';
import { LocalizedDatePipe } from './pipes/localized.datePipe';
import { TranslateModule } from '@ngx-translate/core';
import { HttpClientModule } from '@angular/common/http';
import en from '@angular/common/locales/en';
import jp from '@angular/common/locales/ja';
registerLocaleData(en);
registerLocaleData(jp);
@NgModule({
imports: [
CommonModule,
HttpClientModule,
BrowserModule,
FormsModule,
TranslateModule.forRoot({
defaultLanguage: 'en'
})
],
providers: [DatePipe],
declarations: [AppComponent, LocalizedDatePipe],
bootstrap: [AppComponent]
})
export class AppModule {}
How to use localized Date Pipe
Open the app.component.ts file, import TranslateService and inject this service in constructor for translation.
export class AppComponent {
date_value: string;
constructor(private translate: TranslateService) {}
useLanguage(language: string): void {
this.translate.use(language);
}
ngOnInit() {
this.date_value = new Date().toDateString();
}
}
The below code shows how to use in HTML; add it in the app.component.html file.
<div class="container">
<h2>
Localized Date Pipe Example
</h2>
<div>
<button class="btn-en mr-15" (click)="useLanguage('en')">English</button>
<button class="btn-ja" (click)="useLanguage('ja')">Japanese</button>
</div>
<div class=" result">
{{date_value | localizedDate: 'd MMMM yy, h:mm:ss a' }}
</div>
</div>
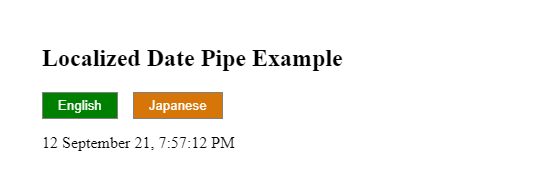
Custom date pipe” ‘localizedDate‘” accepts one parameter “pattern”. We can pass our custom date format or angular predefined date formats as a parameter.
{{ date_Value | localizedDate }}
{{ date_Value | localizedDate:’short’ }}
To change the datetime format we have to pass date time format parameter to the “localizedDate” pipe as shown below
{{ date_Value | localizedDate: ‘hh:mm:ss a’}}
This “localizedDate” is supports all Pre-defined format options of DatePipe
Related Post
Source code available for download
The source code is available on Stackblitz of the localized Date Pipe
Demo Application URL : URL or https://create-localized-datepipe-and-formatting.stackblitz.io