Zoom integration with Angular 12 applications, Zoom provides the Web SDK with video applications powered by Zoom’s core framework inside an Angular framework. In this article, we will demonstrate how Zoom web SDK can be used in Angular application and how to join Zoom meeting from the custom user interface.
Table of Contents
Create an Zoom JWT App in marketplace
In this Step, we create and use a Zoom JWT App in the zoom marketplace to provide API Secrets and API Key for the Zoom web SDK client.
To create a Zoom JWT App
- Sign in to the Zoom Market place click on Develop down menu and choose Build app option.
- Choose JWT app type and click on Create button.
- On the next page, fill out descriptive and contact information.
- Once you’ve accessed your API Key and API Secret and copied over any needed tokens, click Continue.
- On the next step, Choose “Activate your App“.
Set up the Zoom integration with Angular
Before starting Zoom integration with Angular, you first need to create an Angular app. For instructions, on How do I create an Angular project?
Once the Angular project is created, Install the Zoom web SDK npm module.
npm install @zoomus/websdk –save
Create a service class using the following command.
“ng generate service Zoom“
In the ZoomService.ts file, import ZoomMtg and call the preLoadWasm() and prepareJssdk() functions. The following code snippets illustrates how to do generate the meeting signature to authenticate then init, and join the meeting.
import { Injectable } from '@angular/core';
import { ZoomMtg } from '@zoomus/websdk';
import { observable, Observable, of } from 'rxjs';
import { environment } from 'src/environments/environment';
import { SignatureModel, ZoomClientModel } from '../model/zoom.model';
initializeWebSDK(zoomClient: ZoomClientModel): void {
ZoomMtg.preLoadWasm();
ZoomMtg.prepareWebSDK();
ZoomMtg.i18n.load('en-US');
ZoomMtg.i18n.reload('en-US');
ZoomMtg.init({
leaveUrl: environment.leaveUrl,
isSupportAV: true,
success: (success: any) => {
console.log(success)
ZoomMtg.join({
signature: zoomClient.signature,
meetingNumber: zoomClient.meetingNumber,
userName: zoomClient.userName,
apiKey: environment.apiKey,
userEmail: zoomClient.userEmail,
passWord: zoomClient.passWord,
success: (success: any) => {
console.log(success);
},
error: (error: any) => {
console.log(error);
}
})
},
error: (error: any) => {
console.log(error)
}
})
}
genrateSignature(signatureModel: SignatureModel): Observable<string> {
let signature = ZoomMtg.generateSignature({
meetingNumber: signatureModel.meetingNumber,
apiKey: signatureModel.apiKey,
apiSecret: signatureModel.apiSecret,
role: signatureModel.role
})
return of(signature)
}
"assets": [
"src/favicon.ico",
"src/assets",
{
"glob": "**/*",
"input": "./node_modules/@zoomus/websdk/dist/lib/",
"output": "./node_modules/@zoomus/websdk/dist/lib/"
}
],
"styles": [
"./node_modules/@angular/material/prebuilt-themes/indigo-pink.css",
"./node_modules/@zoomus/websdk/dist/css/bootstrap.css",
"./node_modules/@zoomus/websdk/dist/css/react-select.css",
"src/styles.scss"
],
"scripts": [],
"allowedCommonJsDependencies": [
"@zoomus/websdk"
]
Using Zoom Service in Component
To start using our ZoomService within one of StartMeeting components we would first have to import that service and pass the path to that file and then Inject it through the constructor of our component class.
import { Component, OnInit , Inject} from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { Subscription } from 'rxjs';
import { SignatureModel, StartMeeingModel, ZoomClientModel } from 'src/app/model/zoom.model';
import { ZoomService } from 'src/app/services/zoom.service';
import { environment } from 'src/environments/environment';
@Component({
selector: 'app-start-meeting',
templateUrl: './start-meeting.component.html',
styleUrls: ['./start-meeting.component.scss']
})
export class StartMeetingComponent implements OnInit {
startMeetingForm: FormGroup;
subcription: Subscription;
constructor(
private zoomService: ZoomService,
private fb: FormBuilder) {
this.subcription = new Subscription();
}
ngOnInit(): void {
this.initForm();
}
ngOnDestroy() {
this.subcription.unsubscribe();
}
onSubmit() {
if (this.startMeetingForm.valid) {
let formValues: StartMeeingModel = this.startMeetingForm.value;
let signaturePaylod: SignatureModel = {
meetingNumber: formValues.meetingNumber,
apiKey: environment.apiKey,
apiSecret: environment.apiSecret,
role: '0'
};
this.subcription.add(this.zoomService.genrateSignature(signaturePaylod).subscribe((signature: string) => {
this.handleGenrateSignature(signature, formValues);
})
)
}
}
private handleGenrateSignature(signature: string, formValues: StartMeeingModel) {
let meetingPayloads: ZoomClientModel = {
meetingNumber: formValues.meetingNumber.replace(/\s/g, ""),
passWord: formValues.passWord,
signature: signature,
userEmail: formValues.userEmail,
userName: formValues.userName
};
document.getElementById('zmmtg-root')!.style.display = 'block'
this.zoomService.initializeWebSDK(meetingPayloads);
}
private initForm() {
this.startMeetingForm = this.fb.group({
meetingNumber: ['', [Validators.required]],
userName: ['', [Validators.required]],
passWord: ['', [Validators.required]],
userEmail: ['', [Validators.required]]
});
}
}
Click Join Meeting to join the meeting number specified in src/app/start-meeting.component.
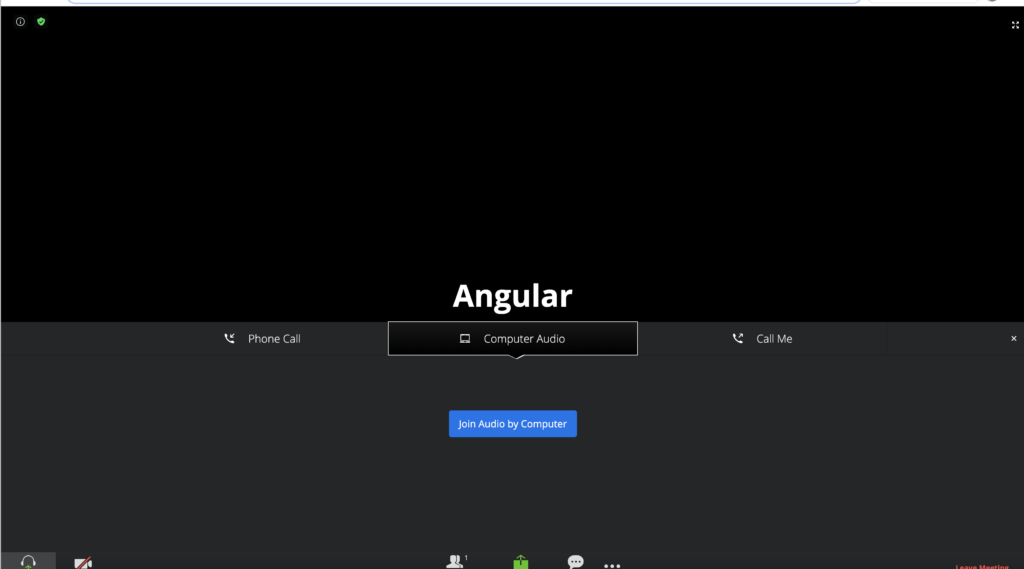
Related Post
Source code available for download
The source code is available on Stackblitz of the Zoom integration with angular 12 | Zoom Meetings into a website.
Demo Application URL : URL or https://create-localized-datepipe-and-formatting.stackblitz.io