In this Angular dynamic search tutorial, we will build a Angular search box for our Angular table that allows users to search the data in their table. We will use the Angular Material Table “filter” method, to provide the functionality of the search, and then we will use Angular to display the search results in our table. We will end with a demo of our completed app.
Table of Contents
How to Create Angular Dynamic Search or Angular Search Box
In this example we will simply create a Angular Dynamic Search enabled table. we will get all data using REST API( for the demo we are using static JSON file). we are using Angular material table and one search box for filter the records in the Table.
In the following code, we have defined the column names to display in the table and I’ll fetch the data from the httpclient. In order to fetch data from our http request, we need to send HTTP request to our http client. Our http client has a function that take request as input and return as output the HTTP Response .
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
displayedColumns: string[] = ['name', 'country', 'currency', 'email', 'pin'];
dataSource = new MatTableDataSource<ICountry>();
keyupSub: Subscription;
countriesSub: Subscription;
searchControl = new FormControl();
constructor(protected _http: HttpClient) {
}
ngOnInit() {
this.getCountries();
}
getCountries() {
this._http.get<ICountry[]>("/assets/country.json").subscribe(res => {
this.dataSource.data = res;
})
}
ngOnDestroy() {
this.keyupSub.unsubscribe();
this.countriesSub.unsubscribe();
}
}
Next, in app.component.html template, we will add an Table component and a input textbox by which a user can filter the records from Table as shown below:
<table mat-table [dataSource]="dataSource" class="mat-elevation-z8" >
<!-- Name Column -->
<ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef> Name </th>
<td mat-cell *matCellDef="let element"> {{element.name}} </td>
</ng-container>
<!-- Email Column -->
<ng-container matColumnDef="email">
<th mat-header-cell *matHeaderCellDef> Email </th>
<td mat-cell *matCellDef="let element"> {{element.email}} </td>
</ng-container>
<!-- Address Column -->
<ng-container matColumnDef="country">
<th mat-header-cell *matHeaderCellDef> Country </th>
<td mat-cell *matCellDef="let element"> {{element.country}} </td>
</ng-container>
<!-- Country Column -->
<ng-container matColumnDef="currency">
<th mat-header-cell *matHeaderCellDef> Currency </th>
<td mat-cell *matCellDef="let element"> {{element.currency}} </td>
</ng-container>
<!-- Pin Column -->
<ng-container matColumnDef="pin">
<th mat-header-cell *matHeaderCellDef> Pin </th>
<td mat-cell *matCellDef="let element"> {{element.pin}} </td>
</ng-container>
<tr mat-header-row *matHeaderRowDef="displayedColumns"></tr>
<tr mat-row *matRowDef="let row; columns: displayedColumns;"></tr>
</table>
All the required steps have been done, now look at the app in your browser. It should look like this:
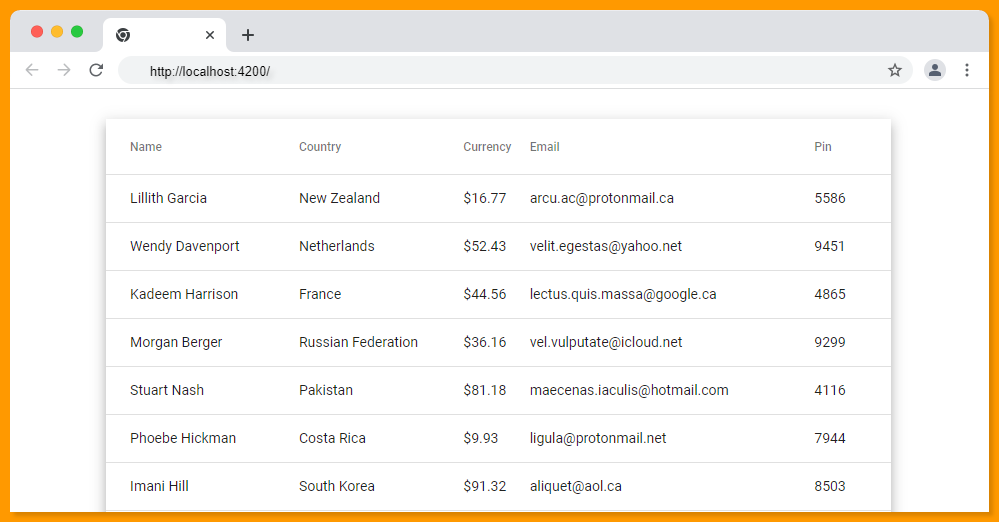
Now we need an input text box to filter the data of the table so that we can filter data easily, we will now need to add a textbox app.component.html.
<mat-form-field appearance="fill" class="txt-full-width">
<mat-label>Search</mat-label>
<input matInput [formControl]="searchControl" placeholder="please enter a value">
</mat-form-field>
We have subscribed to the change event of the textbox and are filtering the table by using typed values.
subSearchBoxChanges() {
this.keyupSub = this.searchControl.valueChanges.pipe(
debounceTime(500)
).subscribe((val: string) => {
this.applyFilter(val);
});
}
applyFilter(filterValue: string): void {
this.dataSource.filter = filterValue;
}
In the above code we are using debounceTime, debounceTime to make sure any fast changes that might happen in the future don’t cause our http request to fire every time. e.g; debounceTime(1000) will wait for 1 second.
Next, Type some text into the Angular search box and see that table being dynamically filtered.
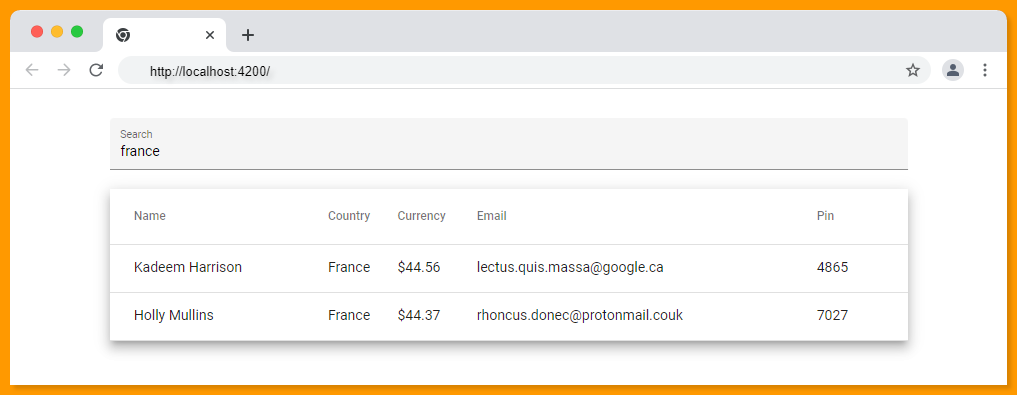
Further Reading
Source code available for download
The source code is available on GitHub for the Angular dynamic search | Angular 12.
URL:https://github.com/decodedscript/angular-material-13-image-upload