Angular Material Table filter by columns is the core feature of the Angular Material table which gives us the flexibility to filter the specific or multiple column( global search) options as per our requirement. In this tutorial, we will create a custom Angular Material Table filter for the table’s values in Angular 11.
Table of Contents
Angular Material Table Filter by Columns or Global Search
In this use case, we will implement Angular Material Table Filter by Columns( global search) functionality in Angular 11 for which we need some data. here we will also create a function to fetch employee data using the HTTP get method from REST API to display data in Angular Material Table.
import { Component } from '@angular/core';
import { MatTableDataSource } from '@angular/material/table';
import { HttpClient } from '@angular/common/http';
import { IEmployee } from './models/IEmployee';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
displayedColumns: string[] = ['name', 'country', 'email', 'address'];
dataSource = new MatTableDataSource<IEmployee>();
constructor(private _http: HttpClient) {
}
ngOnInit() {
this.getEmployeeData();
}
getEmployeeData() {
this._http.get<IEmployee[]>("/assets/data/employee.json").subscribe(res => {
this.dataSource.data = res;
})
}
}
In the above code snippets, we have defined the column header name as a string array which will appear as the column header of the Angular material Table 11.
Next, in the below code snippets, we are filtering the angular Material table data by the value of the textbox.
applyFilter(event: Event): void {
const filter = (event.target as HTMLInputElement).value.trim().toLocaleLowerCase();
this.dataSource.filter = filter;
if (this.dataSource.paginator) {
this.dataSource.paginator.firstPage();
}
}
Next, update the app.component.html template with the below code.
<mat-toolbar color="primary">
<button mat-icon-button>
<mat-icon>menu</mat-icon>
</button>
<span>Angular Material Table Filter By Columns Example</span>
<span class="example-spacer"></span>
</mat-toolbar>
<section class="container">
<mat-form-field appearance="fill">
<mat-label>Search</mat-label>
<input matInput placeholder="please enter a value" (input)="applyFilter($event)">
</mat-form-field>
<table mat-table [dataSource]="dataSource" class="mat-elevation-z8">
<!-- Name Column -->
<ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef> Name </th>
<td mat-cell *matCellDef="let element"> {{element.name}} </td>
</ng-container>
<!-- Email Column -->
<ng-container matColumnDef="email">
<th mat-header-cell *matHeaderCellDef> Email </th>
<td mat-cell *matCellDef="let element"> {{element.email}} </td>
</ng-container>
<!-- Address Column -->
<ng-container matColumnDef="address">
<th mat-header-cell *matHeaderCellDef> address </th>
<td mat-cell *matCellDef="let element"> {{element.address}} </td>
</ng-container>
<!-- Country Column -->
<ng-container matColumnDef="country">
<th mat-header-cell *matHeaderCellDef> Country </th>
<td mat-cell *matCellDef="let element"> {{element.country}} </td>
</ng-container>
<tr mat-header-row *matHeaderRowDef="displayedColumns"></tr>
<tr mat-row *matRowDef="let row; columns: displayedColumns;"></tr>
</table>
</section>
Now, we can check our result of the Angular Table Filter Search:

Angular Material Table Filterpredicate
Let’s get started and implement Filter on Specific Column with filterPredicate by creating a new function “filterTable()” as below
ngOnInit() {
this.getEmployeeData();
this.filterTable();
}
filterTable() {
this.dataSource.filterPredicate = (data: IEmployee, filter: string): boolean => {
return (
data.name.toLocaleLowerCase().includes(filter)
)
}
}
In the above code snippets, the filterPredicate takes the column’s name based on which it filters the table.
As an example, we have just taken the name column, based on if the user type in the search box, it will match only with the value of the column name.
Next, we can check our result of the AngularMaterial Table Filter Search:
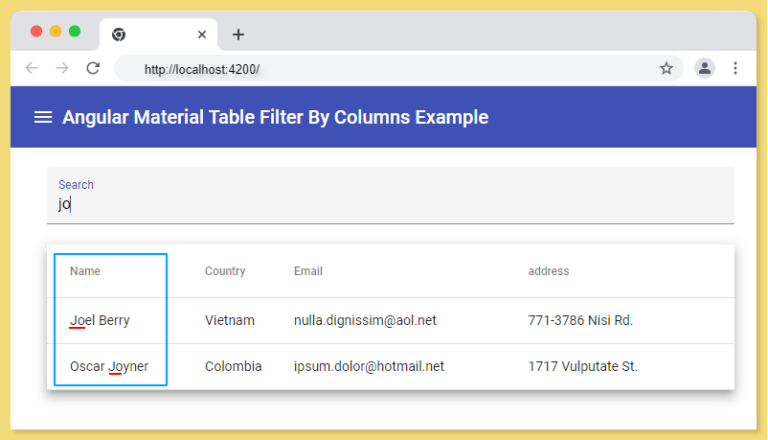
We are using material component, we need to register MatTableModule, MatFormFieldModule, MatInputModule, MatIconModule, MatButtonModule, MatToolbarModule, module in the app.module.ts file.
Source code available for download
The source code is available on GitHub for the Angular Material Table Filter By Columns.
URL:https://github.com/decodedscript/angular-material-13-image-upload