the one-way data binding in Blazor, properties or variables in the @code block of the component are bound with @ symbol to the HTML template block to display value. In this post, we’re going to have a good look at how one-way data binding works in Blazor .Net Core.
Table of Contents
Blazor one-way data binding in .Net Core
In Blazor, The one-way binding provides the ability to take the value of a property or variable and display it on the HTML template. The following are the ways of doing one-way data banding with Blazor.
By using component parameter between components
In the following example, I have done one-way binding by using component parameters between components(User and UserDetails). in the below code snippet, we have a User component that displays a heading, the heading is a one-way bound value the @ symbol followed by the property. When the components are first rendered the headings will be displayed.
@page "/user"
<!-- Parent Component -->
<h1>@pageName child component works!</h1>
<p>you are logged on as a standard user </p>
<UserDetails childTitle="User Details"></UserDetails>
@code {
// input Parameter
private string pageName = String.Empty;
// OnInitializedAsync are invoked when the component is initialized
protected override async Task OnInitializedAsync()
{
pageName = "User";
}
}
In the User component, we are using the UserDetails component to display user details, the User component is passing its title into the UserDetails( child component) via the childTitle component parameter. the complete UserDetails component code and HTML template is as follows.
@page "/user-details"
<!-- Child Component -->
<h3>@childTitle </h3>
<div class="container mt-5">
<div class="row">
<span class="lab-element">Name</span>
<span>@_userDetails.Name</span>
</div>
<div class="row">
<span class="lab-element"> Email</span>
<span>@_userDetails.Email</span>
</div>
<div class="row">
<span class="lab-element"> Phone </span>
<span>@_userDetails.Phone</span>
</div>
</div>
@code {
// input Parameter
[Parameter]
public string childTitle { get; set; }
// user details
private UserDetailsModel _userDetails;
// OnInitializedAsync are invoked when the component is initialized
protected override async Task OnInitializedAsync()
{
// set user details
_userDetails = new UserDetailsModel();
_userDetails.Name = "Alana Barker";
_userDetails.Email = "alana.barker@example.com";
_userDetails.Phone = "000000000000";
}
}
Run the application, the following /user component appears.
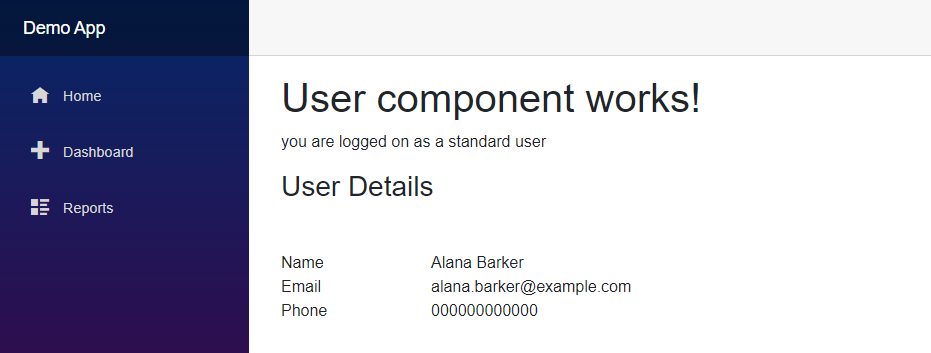
By using model binding
one-way binding can be achieved by using the model binding. In the following example, we have demonstrated an example of model binding. in this example, we have added some properties to UserDetailsModel, as in the following example.
public class UserDetailsModel
{
public string Name { get; set; }
public string Email { get; set; }
public string Phone { get; set; }
public string CompanyName { get; set; }
}
in the following code snippet, OnInitializedAsync method(invoked when the component is initialized) we are setting up the user details.
@code {
private String pageName = String.Empty;
private UserDetailsModel _userDetails;
protected override async Task OnInitializedAsync()
{
// set component heading
pageName = "User Details";
// set user details
_userDetails = new UserDetailsModel();
_userDetails.Name = "Alana Barker";
_userDetails.Email = "alana.barker@example.com";
_userDetails.Phone = "000000000000";
}
}
Next, we need to pass the property name along with @ symbol in ht HTML part of the component.
@page "/user-details"
<h1>@pageName component works!</h1>
<div class="container mt-5">
<div class="row">
<span class="lab-element">Name</span>
<span>@_userDetails.Name</span>
</div>
<div class="row">
<span class="lab-element"> Email</span>
<span>@_userDetails.Email</span>
</div>
<div class="row">
<span class="lab-element"> Phone </span>
<span>@_userDetails.Phone</span>
</div>
</div>
Run the application, the following /user component appears.

By using property binding
Following is an example of a USER component with simpler one-way data binding. The User component displays page heading and the @Code block holds the page heading into the pageName variable which is bound to HTML for display.
@page "/dashboard"
<h1>@pageName component works!</h1>
<p>page description here</p>
@code {
private String pageName =String.Empty;
protected override async Task OnInitializedAsync()
{
pageName="Dashboard";
}
}
Run the application, the following /user component appears.
