Table of Contents
What are we going to build?
In this article, we will do Zoom integration with React JS Application. We will join a Zoom meeting with React JS application and for the configuration that will make a JWT app in Zoom Marketplace. To be able to fit everything into one article, we’ll only set up the basic configuration.
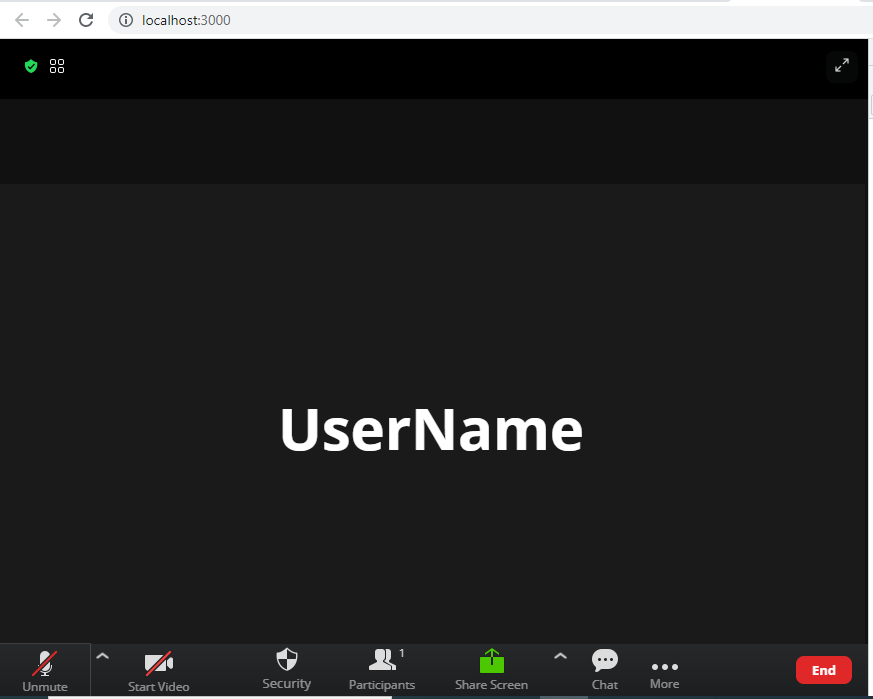
How to create an Zoom JWT App in Marketplace.
Zoom JWT app is required for Zoom integration with React JS application. Zoom also provides OAuth app for integration but in this article, we will cover only Zoom SDK integration using the Zoom JWT app in React JS application. below are the steps to create a Zoom Marketplace app.
- Sign in to the Zoom Market place
- Create JWT app
- Get the API key and API secret
Copy your API KEY and API SECRET and paste them inside the app.js file. API key and Secret will be used to Zoom integration with React js Application.
Zoom integration with React js application
To create a react app we need to run the command npx create-react-app [app name here]. after this command you will get a folder with the same name as you have provided in npx command and next you need to open that folder in Visual Studio Code.
Once the React js project is created, Install the Zoom web SDK npm module with the below NPM command.
npm i @zoomus/websdk
In the component file where you want to use the Web SDK, import ZoomMtg and call the preLoadWasm() and prepareJssdk() functions. These web assemblies download all files that are needed to start a meeting.
ZoomMtg.preLoadWasm();
ZoomMtg.prepareWebSDK();
ZoomMtg.i18n.load('en-US');
ZoomMtg.i18n.reload('en-US');
ZoomMtg.i18n.load(‘en-US’) code is used to set user locale(internationalization in angular ).
Next, the signature is required to start the meeting which we create with API KEY and meeting details. to generate a signature for the Zoom meeting. The following code snippets illustrate how to do generate the meeting signature to authenticate meeting details.
generateSignature = (event) => {
return new Promise((resolve, reject) => {
try {
const signature = ZoomMtg.generateSignature({
meetingNumber: this.state.meetingNumber,
apiKey: this.state.apiKey,
apiSecret: this.state.apiSecret,
role: this.state.role,
});
resolve(signature);
} catch (e) {
reject(Error("generate signature rejected"));
}
});
};
Then we need to modify app.js file. let’s create a handleJoinButton method where we will try to add ZOOM SDK logic.
handleJoinButton = (event) => {
this.generateSignature().then(
(result) => {
// set signature value
this.setState({
signature: result,
});
// initialize zoom web SDK
ZoomMtg.setZoomJSLib("https://source.zoom.us/1.9.9/lib", "/av");
ZoomMtg.preLoadWasm();
ZoomMtg.prepareJssdk();
this.initializeWebSDK();
// display zoom container
document.getElementById("zmmtg-root").style.display = "block";
},
(error) => {
console.log("error");
}
);
};
Then init, and implement the “Join Meeting” functionality.
initializeWebSDK() {
ZoomMtg.init({
leaveUrl: "http://localhost:3000/",
isSupportAV: true,
success: (success) => {
// join meeting - pass meeting details
});
},
error: (error) => {
console.log(error);
},
});
}
Once Zoom SDK has been initialized successfully, add the below code in the success response.
ZoomMtg.join({
signature: this.state.signature,
passWord: this.state.passCode,
meetingNumber: this.state.meetingNumber,
apiKey: this.state.apiKey,
userName: this.state.userName,
userEmail: this.state.userEmail,
success: (success) => {
console.log(success);
},
error: (error) => {
console.log(error);
},
});
Now, let’s put HTML parts into action. We begin by modifying the app.js file, which will include all ZoomSDK functionality. We just make a simple button that calls the function initZoom. Let’s see how this button works.
render() {
return (
<div className="App">
<h2 className="meeting-header">Join Meeting</h2>
<div className="meeting-container">
<div>
<label for="meetingid">Meeting Number</label>
<input
type="text"
id="meetingid"
placeholder="Meeting Number"
value={this.state.meetingNumber}
onChange={this.handleMeetingNumberChange.bind(this)}/>
</div>
<div>
<label for="passcode">Passcode</label>
<input
type="text"
placeholder="Passcode"
value={this.state.passCode}
onChange={this.handlePaascodeChange.bind(this)}/>
</div>
</div>
<div className="action-continer">
<button
className="join-meeting-button"
onClick={this.handleJoinButton}
>
Join Meeting
</button>
</div>
</div>
);
}

We are done with the basic configuration to integrate zoom SDK into the website. now you can start Zoom meetings from the Reactjs application.
Source code available for download
The source code is available on Github of the Zoom integration with React JS application with Example| Integrate Zoom SDK into the website.